- Published on
[NeoX Tutorial] Sending tokens on NeoX with Python
- Authors
- Name
- NeoDashboard
- @DashboardNeo
Description
On this article we will see how to send token on NeoX blockchain in Python.
As an example we will make a simple script allowing us to send 100 $NDMEME token to a particular wallet.
Getting parameters value
For our script we need to gather different information.
Let's go on documentation page to get the following parameters:
- RPC endpoint url
We will also need to get our wallet private key. Let's see how to do It if you are using MetaMask wallet.
Account details
on our Metamask wallet. 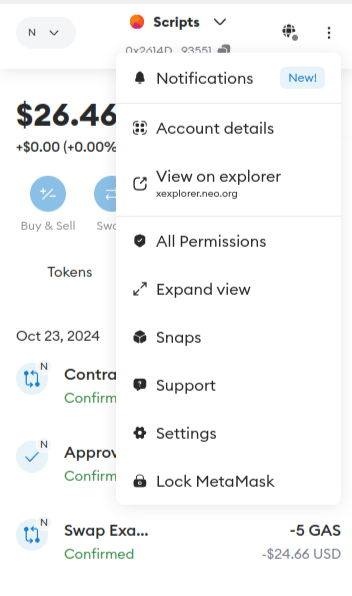
Hold to reveal Private Key
. 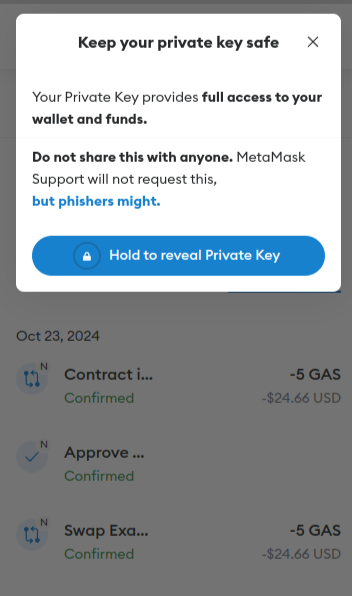
The last thing we will need is the token contract ABI
and contract hash
. If you followed this previous article you already know a way to get It for your desired contract. For our example we will use the default ERC-20 token ABI as our token have been deployed through FTW Smith and isn't verified on NeoX explorer at the time of this article writing. You can find this ABI here and use It with any other token deployed on FTW Smith here.
Python code
Now we have all the information we need and we can switch to the coding step.
Let's start by installing the web3 Python module we will use for interacting with NeoX using the command pip install web3
and then make the script.
The code will contains the following parts. The import of the web3 Python module:
from web3 import Web3
from eth_account import Account
from eth_account.signers.local import LocalAccount
from web3.middleware import ExtraDataToPOAMiddleware
Then the definition of the variable we retrieved previously and we will use for our call:
PK = os.environ.get('NEOX_PK') #Our wallet private key
NDMEME_HASH = "0xE816deE05cf6D0F2a57EB4C489241D8326B5d106"
NDMEME_ABI = open("ndmeme_abi.json").read() #The ABI file
NDMEME_AMOUNT = 100000000000000000000
RPC_ENDPOINT = "https://mainnet-1.rpc.banelabs.org/"
Now we can instantiate a Web3 object that will help connecting to NeoX chain:
w3 = Web3(Web3.HTTPProvider(RPC_ENDPOINT))
account: LocalAccount = Account.from_key(PK)
w3.middleware_onion.inject(ExtraDataToPOAMiddleware, layer=0)
You should note that the last line of this block is important otherwise you won't be able to make web3 module works with NeoX.
Once we have this object we will create the NDMEME contract object:
contract = w3.eth.contract(NDMEME_HASH, abi=NDMEME_ABI)
Now we can build our transaction:
nonce = w3.eth.get_transaction_count(account.address)
tx = contract.functions.transfer(Web3.to_checksum_address(DESTINATION_ADDRESS), NDMEME_AMOUNT).build_transaction({
'from': account.address,
'nonce': nonce
})
Note here the use of Web3.to_checksum_address
method to format the address as It's required on web3 module.
We will now be able to sign and send our transaction:
signed_tx = w3.eth.account.sign_transaction(tx, PK)
tx_hash = w3.eth.send_raw_transaction(signed_tx.raw_transaction)
Now we just have to wait for the transaction to be built in the next block:
w3.eth.wait_for_transaction_receipt(tx_hash.hex())
tx_result = w3.eth.get_transaction_receipt(tx_hash)
print(f"Result for transfer transaction: {tx_result}")
Putting everything together we have the following final script.
import os
from web3 import Web3
from eth_account import Account
from eth_account.signers.local import LocalAccount
from web3.middleware import SignAndSendRawMiddlewareBuilder, ExtraDataToPOAMiddleware
PK = os.environ.get('NEOX_PK')
NDMEME_HASH = "0xE816deE05cf6D0F2a57EB4C489241D8326B5d106"
NDMEME_ABI = open("ndmeme_abi.json").read() #The ABI file
NDMEME_AMOUNT = 100000000000000000000
DESTINATION_ADDRESS = "0x889D02c0df966Ea5BE11dd8E3Eb0d5E4BD0500dD"
RPC_ENDPOINT = "https://mainnet-1.rpc.banelabs.org/"
w3 = Web3(Web3.HTTPProvider(RPC_ENDPOINT))
account: LocalAccount = Account.from_key(PK)
w3.middleware_onion.inject(ExtraDataToPOAMiddleware, layer=0)
contract = w3.eth.contract(NDMEME_HASH, abi=NDMEME_ABI)
nonce = w3.eth.get_transaction_count(account.address)
tx = contract.functions.transfer(Web3.to_checksum_address(DESTINATION_ADDRESS), NDMEME_AMOUNT).build_transaction({
'from': account.address,
'nonce': nonce
})
signed_tx = w3.eth.account.sign_transaction(tx, PK)
tx_hash = w3.eth.send_raw_transaction(signed_tx.raw_transaction)
w3.eth.wait_for_transaction_receipt(tx_hash.hex())
tx_result = w3.eth.get_transaction_receipt(tx_hash)
print(f"Result for transfer transaction: {tx_result}")
Conclusion
You now have an example of Python script to send tokens on NeoX blockchain. Feel free to modify It to your own need and to reach me on Discord if you need more help.