- Published on
[NeoX Tutorial] Interact with The Graph protocol using Python
- Authors
- Name
- NeoDashboard
- @DashboardNeo
Description
End of August Carrot Swap made this announcement saying they completed integration with The Graph. What does this mean? You can now query easily all kind of data from Carrot Swap DEX on NeoX using some simple queries.
In this tutorial we will see how to prepare everything for doing this in a Python script and then make few simple examples.
What is The Graph
The Graph uses GraphQL which is a syntax language that describes how to ask for data. But no worries you don't really need to know about this for this tutorial.
If you want to understand what is The Graph and how It works you can visit their documentation.
Create an account on The Graph
You will first need to create an account here. You can do this by using a Metamask and then enter an email address you will need to use to get a verification code. Once It's done the only thing we need to do is to go on the API Keys tab and create a new that we will use in our Python script
Go to Playground
For understanding how It works and create our first queries we will go on the Carrot Swap The Graph playground page and play a bit with It. By clicking on GraphiQL Explorer icon you can easily click on data you want to retrieve and see the query being written at the same time on the other panel. That's super convenient to start with It. Here is an example of what It looks like.
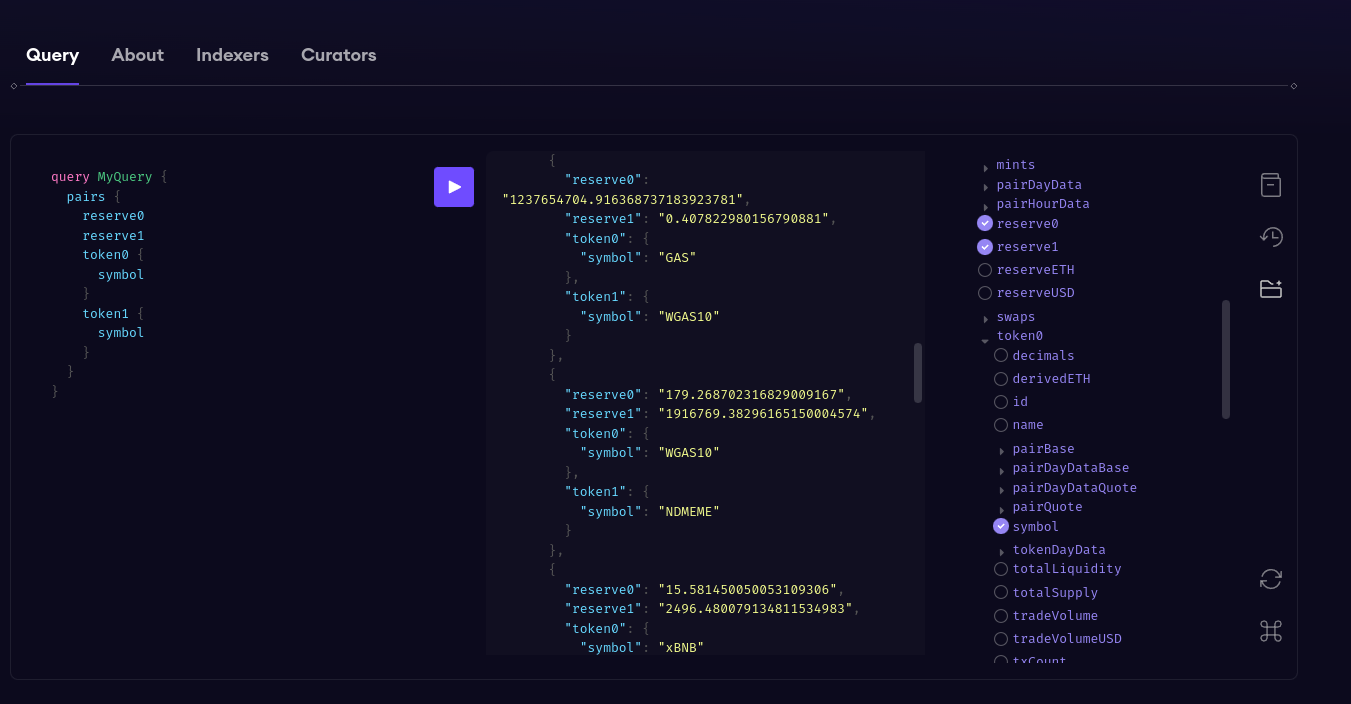
The example in this screenshot is retrieving all the pairs created on Carrot Swap and showing the reserves for those pairs.
Let's code
Creating the Python code now is really simple as It will only requires the API KEY and the GraphQL query we got from the previous steps. Let's do the same thing as in the previous screenshot in Python now.
The code will contains the following parts. The import of the requests Python to make API calls:
import requests
Then the definition of the API_KEY we retrieved previously and we will use for our call:
API_KEY = "THE_GRAPH_API_KEY"
And the definition of the query we got from the playground:
query = """
{
pairs {
reserve0
reserve1
token0 {
symbol
}
token1 {
symbol
}
}
}
"""
And the call to The Graph Carrot Swap subgraph:
res = requests.post(f'https://gateway.thegraph.com/api/{API_KEY}/subgraphs/id/DVjqbErAXc9WDFMaZ6nwrH3GUqtH4vfRdPFar2uGtu12', json={'query': query})
Putting everything together and setting the key as an environment variable we have the following final script.
import os
import requests
API_KEY = os.environ.get("THE_GRAPH_API_KEY")
query = """
{
pairs {
reserve0
reserve1
token0 {
symbol
}
token1 {
symbol
}
}
}
"""
res = requests.post(f'https://gateway.thegraph.com/api/{API_KEY}/subgraphs/id/DVjqbErAXc9WDFMaZ6nwrH3GUqtH4vfRdPFar2uGtu12', json={'query': query})
print(res.json())
Another example
Once we know how to build queries It's easy to make new ones.
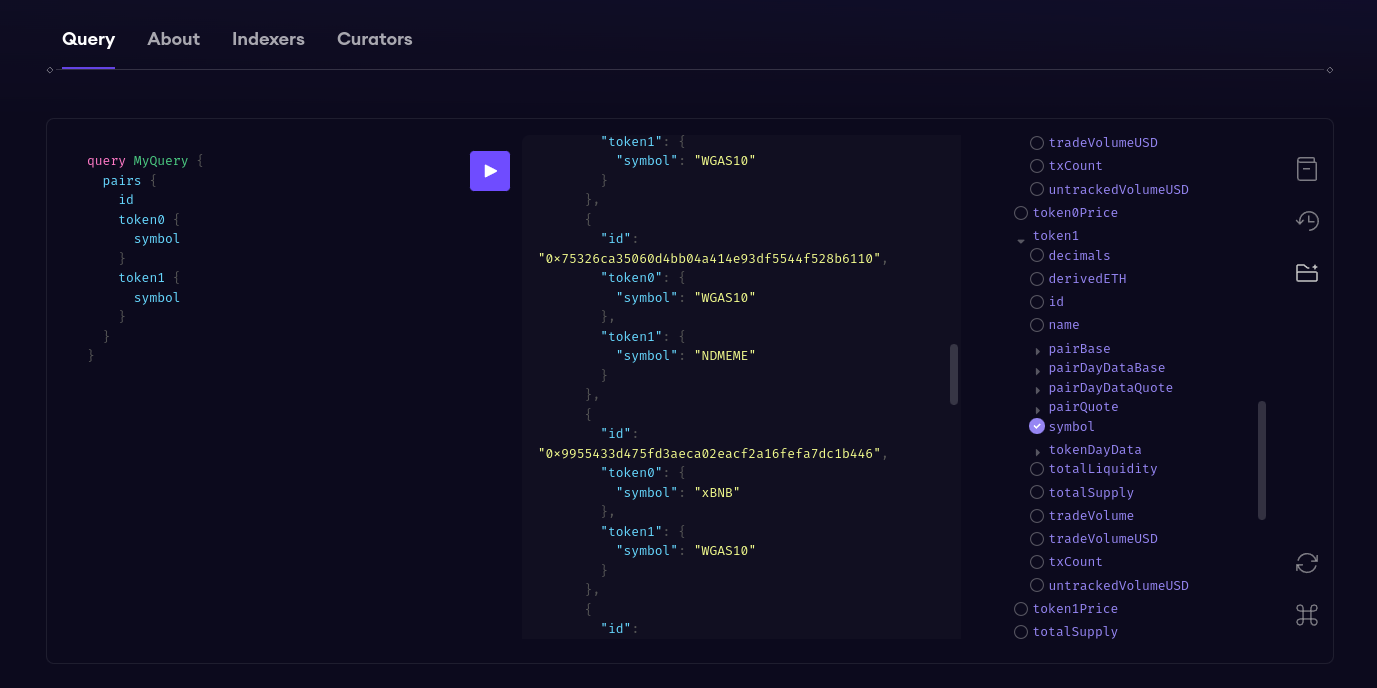
import os
import requests
API_KEY = os.environ.get("THE_GRAPH_API_KEY")
query = """
{
swaps(where: {pair_: {id: "0x75326ca35060d4bb04a414e93df5544f528b6110"}}) {
id
transaction {
id
}
amountUSD
}
}
"""
res = requests.post(f'https://gateway.thegraph.com/api/{API_KEY}/subgraphs/id/DVjqbErAXc9WDFMaZ6nwrH3GUqtH4vfRdPFar2uGtu12', json={'query': query})
swaps_usd_total = sum([float(x['amountUSD']) for x in res.json()['data']['swaps']])
print(swaps_usd_total)
Conclusion
You now have an example of Python script to interact with Carrot Swap subgraph on The Graph. Feel free to modify It to your own need and to reach me on Discord if you need more help.
If you want to see a video of using The Graph playground you can watch this one on my YouTube channel